Chess---->簡單命令框象棋(人VS人),chess----vs
簡單粗暴,直接先上代碼:
ChessBoard.h:
1 #ifndef CHESBOARD_H
2 #include<iostream>
3 #include<string>
4 using namespace std;
5 class Chess
6 {
7 public:
8 virtual string getName() { return name; }
9 //virtual bool judge(int a, int b, int c, int d);
10 Chess(string name, int color) :name(name), color(color) {}
11 virtual int judge(int a, int b, int c, int d) { return 4; } //1 移動非法 2 可移動到空位子 3 吃子
12 virtual int getColor() { return color; }
13 protected:
14 string name;
15 int color;
16 };
17
18 class Shuai :public Chess
19 {
20 public:
21 Shuai(string a, int b) :Chess(a, b) {} //a 名字 b 顏色
22 int judge(int a, int b, int c, int d);
23 };
24
25 class Shi :public Chess
26 {
27 public:
28 Shi(string a, int b) :Chess(a, b) {}
29 int judge(int a, int b, int c, int d);
30 };
31
32 class Bing :public Chess
33 {
34 public:
35 Bing(string name, int color) :Chess(name, color) {}
36 int judge(int a, int b, int c, int d);
37 };
38
39 class Xiang :public Chess
40 {
41 public:
42 Xiang(string name, int color) :Chess(name, color) {}
43 int judge(int a, int b, int c, int d);
44 };
45
46 class Ma :public Chess
47 {
48 public:
49 int judge(int a, int b, int c, int d);
50 Ma(string name, int color) :Chess(name, color) {}
51 };
52
53
54 class Pao :public Chess
55 {
56 public:
57 int judge(int a, int b, int c, int d);
58 Pao(string name, int color) :Chess(name, color) {}
59 };
60 class Che :public Chess
61 {
62 public:
63 int judge(int a, int b, int c, int d);
64 Che(string name, int color) :Chess(name, color) {}
65 };
66
67 class Player
68 {
69 public:
70 Player(int a) :color(a) {}
71 bool judge(int a, int b);
72 void inPut();
73 void moveChess(int a, int b, int c, int d);
74 void eatChess(int a, int b, int c, int d);
75 private:
76 int color;
77 };
78 #endif // CHESBOARD_H
Chess:
1 #include"ChesBoard.h"
2 #include<Windows.h>
3 #include<vector>
4
5 class ChessBoard {
6 public:
7 vector<vector<Chess *> > v_position;
8 bool judgePositionIsNULL(int a, int b) { //判斷棋盤上是否是空位
9 if (v_position[a][b]) {
10 return false;
11 }
12 return true;
13 }
14
15 int judgePositionIsColor(int a, int b) { //有子則返回顏色(1為紅色,2為黑色)
16 return v_position[a][b]->getColor();
17 }
18 ChessBoard() {
19 for (int i = 0; i < 10; i++) {
20 vector<Chess *> v_cell(9, NULL);
21 v_position.push_back(v_cell);
22 }
23 }
24 void init_Red();
25 void init_Black();
26 void showBoard();
27 };
28
29 ChessBoard cb;
30
31 //靜態數據,紅方
32 Shuai shuai_red("帥", 1);
33 Shi shi_red1("士", 1);
34 Shi shi_red2("士", 1);
35 Xiang xiang_red1("相", 1);
36 Xiang xiang_red2("相", 1);
37 Ma ma_red1("馬", 1);
38 Ma ma_red2("馬", 1);
39 Che che_red1("車", 1);
40 Che che_red2("車", 1);
41 Pao pao_red1("炮", 1);
42 Pao pao_red2("炮", 1);
43 Bing bing_red1("兵", 1);
44 Bing bing_red2("兵", 1);
45 Bing bing_red3("兵", 1);
46 Bing bing_red4("兵", 1);
47 Bing bing_red5("兵", 1);
48
49 //靜態數據,黑方
50 Shuai shuai_black("將", 2);
51 Shi shi_black1("仕", 2);
52 Shi shi_black2("仕", 2);
53 Xiang xiang_black1("相", 2);
54 Xiang xiang_black2("相", 2);
55 Ma ma_black1("馬", 2);
56 Ma ma_black2("馬", 2);
57 Che che_black1("車", 2);
58 Che che_black2("車", 2);
59 Pao pao_black1("炮", 2);
60 Pao pao_black2("炮", 2);
61 Bing bing_black1("卒", 2);
62 Bing bing_black2("卒", 2);
63 Bing bing_black3("卒", 2);
64 Bing bing_black4("卒", 2);
65 Bing bing_black5("卒", 2);
66
67
68 void ChessBoard::init_Red() {
69 v_position[0][4] = &shuai_red;
70 v_position[0][3] = &shi_red1;
71 v_position[0][5] = &shi_red2;
72 v_position[0][2] = &xiang_red1;
73 v_position[0][6] = &xiang_red2;
74 v_position[0][1] = &ma_red1;
75 v_position[0][7] = &ma_red2;
76 v_position[0][0] = &che_red1;
77 v_position[0][8] = &che_red2;
78 v_position[2][1] = &pao_red1;
79 v_position[2][7] = &pao_red2;
80 v_position[3][0] = &bing_red1;
81 v_position[3][2] = &bing_red2;
82 v_position[3][4] = &bing_red3;
83 v_position[3][6] = &bing_red4;
84 v_position[3][8] = &bing_red5;
85 }
86
87 void ChessBoard::init_Black() {
88 v_position[9][4] = &shuai_black;
89 v_position[9][3] = &shi_black1;
90 v_position[9][5] = &shi_black2;
91 v_position[9][2] = &xiang_black1;
92 v_position[9][6] = &xiang_black2;
93 v_position[9][1] = &ma_black1;
94 v_position[9][7] = &ma_black2;
95 v_position[9][0] = &che_black1;
96 v_position[9][8] = &che_black2;
97 v_position[7][1] = &pao_black1;
98 v_position[7][7] = &pao_black2;
99 v_position[6][0] = &bing_black1;
100 v_position[6][2] = &bing_black2;
101 v_position[6][4] = &bing_black3;
102 v_position[6][6] = &bing_black4;
103 v_position[6][8] = &bing_black5;
104 }
105
106 void ChessBoard::showBoard() {
107 //用於記錄已打印的行數,以便在准確位置打印“楚河、漢界”
108 int flag = 0;
109 cout << " ";
110 for (int i = 0; i < 9; i++) {
111 cout << ' ' << i << " ";
112 }
113 cout << endl;
114 cout << " ------------------------------------" << endl;
115 for (auto row : v_position) {
116 cout << flag << '|' << ' ';
117 flag++;
118 for (auto column : row) {
119 if (column == NULL)
120 cout << " 十 ";
121 else {
122 if (column->getColor() == 1) {
123 SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), FOREGROUND_INTENSITY | FOREGROUND_RED);
124 }
125 else {
126 SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), FOREGROUND_INTENSITY | FOREGROUND_BLUE);
127 }
128 cout << ' ';
129 cout << (column->getName());
130 cout << ' ';
131 SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE), FOREGROUND_INTENSITY);
132 }
133 }
134 cout << endl << " |" << endl;
135 if (flag == 5) {
136 cout << " ------------------------------------" << endl;
137 cout << " 楚河 漢界 " << endl;
138 cout << " ------------------------------------" << endl;
139 cout << endl;
140 }
141 }
142 }
143 int Shuai::judge(int a, int b, int c, int d)
144 {
145 if (getName() == "帥") //判斷帥還是將
146 {
147 if ((c <= 2 && c >= 0) && (d >= 3 && d <= 5)) // 帥只能在中心9格移動
148 {
149 if (cb.judgePositionIsNULL(c, d)) //判斷在將要移動到的地方是否有子
150 {
151 if ((c == a&&d == b + 1) || (c == a&&d == b - 1) || (c == a + 1 && d == b) || (c == a - 1 && d == b)) //判斷移動是否為一格
152 {
153 return 2;
154 }
155 }
156 else
157 {
158 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
159 {
160 return 1;
161 }
162 else //若是黑子,返回吃子判斷
163 {
164 return 3;
165 }
166 }
167 }
168 //此時可能對將(飛將)
169 else if((c >= 0 && c <= 9) && (d >= 0 && d <= 8) && b == d && cb.v_position[c][d]){
170 if (cb.v_position[c][d]->getName() == "將") {
171 for (int i = a + 1; i < c; i++)
172 //中間有子,則不能移動,返回1
173 if (cb.v_position[i][b])
174 return 1;
175 return 3;
176 }
177 }
178 }
179 if (getName() == "將") //判斷帥還是將
180 {
181 if ((c <= 9 && c >= 7) && (d >= 3 && d <= 5)) // 將只能在中心9格移動
182 {
183 if (cb.judgePositionIsNULL(c, d)) //判斷在將要移動到的地方是否有己方的子
184 {
185 if ((c == a&&d == b + 1) || (c == a&&d == b - 1) || (c == a + 1 && d == b) || (c == a - 1 && d == b)) //判斷移動是否為一格
186 {
187 return 2;
188 }
189 }
190 else
191 {
192 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑
193 {
194 return 1;
195 }
196 else //若是紅子,返回吃子判斷
197 {
198 return 3;
199 }
200 }
201 }
202 //此時可能對將(飛將)
203 else if ((c >= 0 && c <= 9) && (d >= 0 && d <= 8) && b == d && cb.v_position[c][d]) {
204 if (cb.v_position[c][d]->getName() == "帥") {
205 for (int i = a - 1; i > c; i--)
206 //中間有子,則不能移動,返回1
207 if (cb.v_position[i][b])
208 return 1;
209 return 3;
210 }
211 }
212 }
213 return 1;
214 }
215
216 int Shi::judge(int a, int b, int c, int d)
217 {
218 if (cb.judgePositionIsColor(a, b) == 1) //判斷是紅子
219 {
220 if ((c <= 2 && c >= 0) && (d >= 3 && d <= 5)) //判斷目的地是否在中心9格
221 {
222 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
223 {
224 if ((c == a + 1 && d == b + 1) || (c == a + 1 && d == b - 1) || (c == a - 1 && d == b + 1) || (c == a - 1 && d == b - 1)) //判斷是否符合移動規則
225 {
226 return 2;
227 }
228 }
229 else
230 {
231 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
232 {
233 return 1;
234 }
235 else //若是黑子,返回吃子判斷
236 {
237 return 3;
238 }
239 }
240 }
241 }
242 if (cb.judgePositionIsColor(a, b) == 2) //判斷是黑子
243 {
244 if ((c <= 9 && c >= 7) && (d >= 3 && d <= 5)) //判斷目的地是否在中心9格
245 {
246 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
247 {
248 if ((c == a + 1 && d == b + 1) || (c == a + 1 && d == b - 1) || (c == a - 1 && d == b + 1) || (c == a - 1 && d == b - 1)) //判斷是否符合移動規則
249 {
250 return 2;
251 }
252 }
253 else
254 {
255 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑 判斷是哪方子
256 {
257 return 1;
258 }
259 else //若是紅子,返回吃子判斷
260 {
261 return 3;
262 }
263 }
264 }
265 }
266 return 1;
267 }
268 int Bing::judge(int a, int b, int c, int d)
269 {
270 if (cb.judgePositionIsColor(a, b) == 1) //判斷是紅子
271 {
272 if (a >= 5) //判斷是否過河界
273 {
274 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
275 {
276 if ((c == a&&d == b + 1) || (c == a&&d == b - 1) || (c == a + 1 && d == b))
277 {
278 return 2;
279 }
280 }
281 else
282 {
283 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
284 {
285 return 1;
286 }
287 else //若是黑子,返回吃子判斷
288 {
289 return 3;
290 }
291 }
292 }
293 else //未過河界
294 {
295 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
296 {
297 if (c == a + 1 && d == b)
298 {
299 return 2;
300 }
301 }
302 else
303 {
304 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
305 {
306 return 1;
307 }
308 else //若是黑子,返回吃子判斷
309 {
310 return 3;
311 }
312 }
313 }
314 }
315 if (cb.judgePositionIsColor(a, b) == 2) //判斷是黑子
316 {
317 if (a <= 4) //判斷是否過河界
318 {
319 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
320 {
321 if ((c == a&&d == b + 1) || (c == a&&d == b - 1) || (c == a - 1 && d == b))
322 {
323 return 2;
324 }
325 }
326 else
327 {
328 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑 判斷是哪方子
329 {
330 return 1;
331 }
332 else //若是紅子,返回吃子判斷
333 {
334 return 3;
335 }
336 }
337 }
338 else //未過河界
339 {
340 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
341 {
342 if (c == a - 1 && d == b)
343 {
344 return 2;
345 }
346 }
347 else
348 {
349 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑 判斷是哪方子
350 {
351 return 1;
352 }
353 else //若是紅子,返回吃子判斷
354 {
355 return 3;
356 }
357 }
358 }
359 }
360 return 1;
361 }
362 int Xiang::judge(int a, int b, int c, int d)
363 {
364 if (cb.judgePositionIsColor(a, b) == 1) //判斷是紅子
365 {
366 if (b - 1 >= 0 && a - 1 >= 0 && !cb.judgePositionIsNULL(a - 1, b - 1)) //判斷是否別象腿左上方 數組越界問題
367 {
368 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
369 {
370 if ((c == a - 2 && d == b + 2) || (c == a + 2 && d == b + 2) || (c == a + 2 && d == b - 2)) //判斷是否符合移動規則
371 return 2;
372 }
373 else
374 {
375 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
376 {
377 return 1;
378 }
379 else //若是黑子,返回吃子判斷
380 {
381 return 3;
382 }
383 }
384 }
385 else if (b + 1 <= 9 && a - 1 >= 0 && !cb.judgePositionIsNULL(a - 1, b + 1)) //判斷是否別象腿右上方 數組越界問題
386 {
387 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
388 {
389 if ((c == a + 2 && d == b + 2) || (c == a + 2 && d == b - 2) || (c == a - 2 && d == b - 2)) //判斷是否符合移動規則
390 return 2;
391 }
392 else
393 {
394 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
395 {
396 return 1;
397 }
398 else //若是黑子,返回吃子判斷
399 {
400 return 3;
401 }
402 }
403 }
404 else if (a + 1 <= 9 && b - 1 >= 0 && !cb.judgePositionIsNULL(a + 1, b - 1)) //判斷是否別象腿左下方 數組越界問題
405 {
406 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
407 {
408 if ((c == a - 2 && d == b - 2) || (c == a - 2 && d == b + 2) || (c == a + 2 && d == b + 2)) //判斷是否符合移動規則
409 return 2;
410 }
411 else
412 {
413 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
414 {
415 return 1;
416 }
417 else //若是黑子,返回吃子判斷
418 {
419 return 3;
420 }
421 }
422 }
423 else if (a + 1 <= 9 && b + 1 <= 9 && !cb.judgePositionIsNULL(a + 1, b + 1)) //判斷是否別象腿右下方 數組越界問題
424 {
425 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
426 {
427 if ((c == a - 2 && d == b + 2) || (c == a - 2 && d == b - 2) || (c == a + 2 && d - 2)) //判斷是否符合移動規則
428 return 2;
429 }
430 else
431 {
432 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
433 {
434 return 1;
435 }
436 else //若是黑子,返回吃子判斷
437 {
438 return 3;
439 }
440 }
441 }
442 else
443 {
444 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
445 {
446 if ((c == a - 2 && d == b + 2) || (c == a - 2 && d == b - 2) || (c == a + 2 && d == b - 2) || (c == a + 2 && d == b + 2)) //判斷是否符合移動規則
447 return 2;
448 }
449 else
450 {
451 if (cb.judgePositionIsColor(c, d) == 1) //1紅 2黑 判斷是哪方子
452 {
453 return 1;
454 }
455 else //若是黑子,返回吃子判斷
456 {
457 return 3;
458 }
459 }
460 }
461 }
462 if (cb.judgePositionIsColor(a, b) == 2) //判斷是黑子
463 {
464 if (b - 1 >= 0 && a - 1 >= 0 && !cb.judgePositionIsNULL(a - 1, b - 1)) //判斷是否別象腿左上方 數組越界問題
465 {
466 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
467 {
468 if ((c == a - 2 && d == b + 2) || (c == a + 2 && d == b + 2) || (c == a + 2 && d == b - 2)) //判斷是否符合移動規則
469 return 2;
470 }
471 else
472 {
473 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑 判斷是哪方子
474 {
475 return 1;
476 }
477 else //若是紅子,返回吃子判斷
478 {
479 return 3;
480 }
481 }
482 }
483 else if (b + 1 <= 9 && a - 1 >= 0 && !cb.judgePositionIsNULL(a - 1, b + 1)) //判斷是否別象腿右上方 數組越界問題
484 {
485 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
486 {
487 if ((c == a + 2 && d == b + 2) || (c == a + 2 && d == b - 2) || (c == a - 2 && d == b - 2)) //判斷是否符合移動規則
488 return 2;
489 }
490 else
491 {
492 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑 判斷是哪方子
493 {
494 return 1;
495 }
496 else //若是紅子,返回吃子判斷
497 {
498 return 3;
499 }
500 }
501 }
502 else if (a + 1 <= 9 && b - 1 >= 0 && !cb.judgePositionIsNULL(a + 1, b - 1)) //判斷是否別象腿左下方 數組越界問題
503 {
504 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
505 {
506 if ((c == a - 2 && d == b - 2) || (c == a - 2 && d == b + 2) || (c == a + 2 && d == b + 2)) //判斷是否符合移動規則
507 return 2;
508 }
509 else
510 {
511 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑 判斷是哪方子
512 {
513 return 1;
514 }
515 else //若是紅子,返回吃子判斷
516 {
517 return 3;
518 }
519 }
520 }
521 else if (a + 1 <= 9 && b + 1 <= 9 && !cb.judgePositionIsNULL(a + 1, b + 1)) //判斷是否別象腿右下方 數組越界問題
522 {
523 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
524 {
525 if ((c == a - 2 && d == b + 2) || (c == a - 2 && d == b - 2) || (c == a + 2 && d - 2)) //判斷是否符合移動規則
526 return 2;
527 }
528 else
529 {
530 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑 判斷是哪方子
531 {
532 return 1;
533 }
534 else //若是紅子,返回吃子判斷
535 {
536 return 3;
537 }
538 }
539 }
540 else
541 {
542 if (cb.judgePositionIsNULL(c, d)) //判斷目的地是否有子
543 {
544 if ((c == a - 2 && d == b + 2) || (c == a - 2 && d == b - 2) || (c == a + 2 && d == b - 2) || (c == a + 2 && d == b + 2)) //判斷是否符合移動規則
545 return 2;
546 }
547 else
548 {
549 if (cb.judgePositionIsColor(c, d) == 2) //1紅 2黑 判斷是哪方子
550 {
551 return 1;
552 }
553 else //若是紅子,返回吃子判斷
554 {
555 return 3;
556 }
557 }
558 }
559 }
560 return 1;
561 }
562
563 int Che::judge(int a, int b, int c, int d) {
564
565 //判斷目標點是否在棋盤界內
566 if ((c >= 0 && c <= 9) && (d >= 0 && d <= 8)) {
567
568 //判斷車是否走的是直線,否則不能移動,返回1
569 if (a == c || b == d) {
570
571 if (a == c) { //橫向移動
572 //目標點是自身,則不能移動,返回1
573 if (b == d) {
574 return 1;
575 }
576 else if (b > d) {
577 for (int i = b - 1; i > d; i--)
578 //中間有子,則不能移動,返回1
579 if (cb.v_position[c][i])
580 return 1;
581 }
582 else {
583 for (int i = b + 1; i < d; i++)
584 //中間有子,則不能移動,返回1
585 if (cb.v_position[c][i])
586 return 1;
587 }
588 //目標點是空位置,則可直接移到目標點
589 if (!cb.v_position[c][d]) {
590 return 2;
591 }
592 else {
593 //目標位置是己方棋子,則不能移動,返回1
594 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
595 return 1;
596 }
597 else {
598 return 3;
599 }
600 }
601 }
602 else { //縱向移動
603 //目標點是自身,則不能移動,返回1
604 if (a == c) {
605 return 1;
606 }
607 else if (a > c) {
608 for (int i = a - 1; i > c; i--)
609 //中間有子,則不能移動,返回1
610 if (cb.v_position[i][d])
611 return 1;
612 }
613 else {
614 for (int i = a + 1; i < c; i++)
615 //中間有子,則不能移動,返回1
616 if (cb.v_position[i][d])
617 return 1;
618 }
619 //目標點是空位置,則可直接移到目標點
620 if (!cb.v_position[c][d]) {
621 return 2;
622 }
623 else {
624 //目標位置是己方棋子,則不能移動,返回1
625 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
626 return 1;
627 }
628 else {
629 return 3;
630 }
631 }
632 }
633 }
634 else {
635 return 1;
636 }
637 }
638 else {
639 return 1;
640 }
641
642 }
643
644 int Ma::judge(int a, int b, int c, int d) {
645 //判斷目標點是否在棋盤界內
646 if ((c >= 0 && c <= 9) && (d >= 0 && d <= 8)) {
647 //判斷走的是否是“日”
648 if (((a - c)*(a - c) + (b - d)*(b - d)) != 5) {
649 return 1;
650 }
651 else {
652 //偏左右
653 if (abs(a - c) == 1) {
654 //上
655 if (a > c) {
656 if (b > d && !cb.v_position[a][b - 1]/*判斷是否別馬腳*/) {
657 //目標點是空位置,則可直接移到目標點
658 if (!cb.v_position[c][d]) {
659 return 2;
660 }
661 else {
662 //目標位置是己方棋子,則不能移動,返回1
663 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
664 return 1;
665 }
666 else {
667 return 3;
668 }
669 }
670 }
671 else if (b < d && !cb.v_position[a][b + 1]/*判斷是否別馬腳*/) {
672 //目標點是空位置,則可直接移到目標點
673 if (!cb.v_position[c][d]) {
674 return 2;
675 }
676 else {
677 //目標位置是己方棋子,則不能移動,返回1
678 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
679 return 1;
680 }
681 else {
682 return 3;
683 }
684 }
685 }
686 //別馬腳了,不能移動
687 else {
688 return 1;
689 }
690 }
691 //下
692 else {
693 if (b > d && !cb.v_position[a][b - 1]/*判斷是否別馬腳*/) {
694 //目標點是空位置,則可直接移到目標點
695 if (!cb.v_position[c][d]) {
696 return 2;
697 }
698 else {
699 //目標位置是己方棋子,則不能移動,返回1
700 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
701 return 1;
702 }
703 else {
704 return 3;
705 }
706 }
707 }
708 else if (b < d && !cb.v_position[a][b + 1]/*判斷是否別馬腳*/) {
709 //目標點是空位置,則可直接移到目標點
710 if (!cb.v_position[c][d]) {
711 return 2;
712 }
713 else {
714 //目標位置是己方棋子,則不能移動,返回1
715 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
716 return 1;
717 }
718 else {
719 return 3;
720 }
721 }
722 }
723 //別馬腳了,不能移動
724 else {
725 return 1;
726 }
727 }
728 }
729 //偏上下
730 else {
731 //左
732 if (b > d) {
733 if (a > c && !cb.v_position[a - 1][b]/*判斷是否別馬腳*/) {
734 //目標點是空位置,則可直接移到目標點
735 if (!cb.v_position[c][d]) {
736 return 2;
737 }
738 else {
739 //目標位置是己方棋子,則不能移動,返回1
740 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
741 return 1;
742 }
743 else {
744 return 3;
745 }
746 }
747 }
748 else if (a < c && !cb.v_position[a + 1][b]/*判斷是否別馬腳*/) {
749 //目標點是空位置,則可直接移到目標點
750 if (!cb.v_position[c][d]) {
751 return 2;
752 }
753 else {
754 //目標位置是己方棋子,則不能移動,返回1
755 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
756 return 1;
757 }
758 else {
759 return 3;
760 }
761 }
762 }
763 //別馬腳了,不能移動
764 else {
765 return 1;
766 }
767 }
768 //右
769 else {
770 if (a > c && !cb.v_position[a - 1][b]/*判斷是否別馬腳*/) {
771 //目標點是空位置,則可直接移到目標點
772 if (!cb.v_position[c][d]) {
773 return 2;
774 }
775 else {
776 //目標位置是己方棋子,則不能移動,返回1
777 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
778 return 1;
779 }
780 else {
781 return 3;
782 }
783 }
784 }
785 else if (a < c && !cb.v_position[a + 1][b]/*判斷是否別馬腳*/) {
786 //目標點是空位置,則可直接移到目標點
787 if (!cb.v_position[c][d]) {
788 return 2;
789 }
790 else {
791 //目標位置是己方棋子,則不能移動,返回1
792 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
793 return 1;
794 }
795 else {
796 return 3;
797 }
798 }
799 }
800 //別馬腳了,不能移動
801 else {
802 return 1;
803 }
804 }
805 }
806 }
807 }
808 else {
809 return 1;
810 }
811 }
812
813 int Pao::judge(int a, int b, int c, int d) {
814 //用於標記炮中間是否隔子
815 int flag;
816 //判斷目標點是否在棋盤界內
817 if ((c >= 0 && c <= 9) && (d >= 0 && d <= 8)) {
818 //判斷炮是否走的是直線,否則不能移動,返回1
819 if (a == c || b == d) {
820 if (a == c) { //橫向移動
821 //目標點是自身,則不能移動,返回1
822 if (b == d) {
823 return 1;
824 }
825 else if (b > d) {
826 flag = 0;
827 for (int i = b - 1; i > d; i--) {
828 //中間有一子,flag++
829 if (cb.v_position[c][i])
830 flag++;
831 }
832 switch (flag) {
833 case 0:
834 //目標點是空位置,則可直接移到目標點
835 if (!cb.v_position[c][d]) {
836 return 2;
837 }
838 else {
839 //目標位置不為空,則不能移動
840 return 1;
841 }
842 break;
843 case 1:
844 //目標點是空位置,則炮無法隔一子移動
845 if (!cb.v_position[c][d]) {
846 return 1;
847 }
848 else {
849 //目標位置是己方棋子,則不能吃,返回1
850 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
851 return 1;
852 }
853 else {
854 return 3;
855 }
856 }
857 break;
858 default:
859 return 1;
860
861 }
862
863 }
864 else {
865 flag = 0;
866 for (int i = b + 1; i < d; i++) {
867 //中間有一子,flag++
868 if (cb.v_position[c][i])
869 flag++;
870 }
871 switch (flag) {
872 case 0:
873 //目標點是空位置,則可直接移到目標點
874 if (!cb.v_position[c][d]) {
875 return 2;
876 }
877 else {
878 //目標位置不為空,則不能移動
879 return 1;
880 }
881 break;
882 case 1:
883 //目標點是空位置,則炮無法隔一子移動
884 if (!cb.v_position[c][d]) {
885 return 1;
886 }
887 else {
888 //目標位置是己方棋子,則不能吃,返回1
889 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
890 return 1;
891 }
892 else {
893 return 3;
894 }
895 }
896 break;
897 default:
898 return 1;
899
900 }
901 }
902 }
903 else { //縱向移動
904 //目標點是自身,則不能移動,返回1
905 if (a == c) {
906 return 1;
907 }
908 else if (a > c) {
909 flag = 0;
910 for (int i = a - 1; i > c; i--) {
911 //中間有一子,flag++
912 if (cb.v_position[i][d])
913 flag++;
914 }
915 switch (flag) {
916 case 0:
917 //目標點是空位置,則可直接移到目標點
918 if (!cb.v_position[c][d]) {
919 return 2;
920 }
921 else {
922 //目標位置不為空,則不能移動
923 return 1;
924 }
925 break;
926 case 1:
927 //目標點是空位置,則炮無法隔一子移動
928 if (!cb.v_position[c][d]) {
929 return 1;
930 }
931 else {
932 //目標位置是己方棋子,則不能吃,返回1
933 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
934 return 1;
935 }
936 else {
937 return 3;
938 }
939 }
940 break;
941 default:
942 return 1;
943
944 }
945
946 }
947 else {
948 flag = 0;
949 for (int i = a + 1; i < c; i++) {
950 //中間有一子,flag++
951 if (cb.v_position[i][d])
952 flag++;
953 }
954 switch (flag) {
955 case 0:
956 //目標點是空位置,則可直接移到目標點
957 if (!cb.v_position[c][d]) {
958 return 2;
959 }
960 else {
961 //目標位置不為空,則不能移動
962 return 1;
963 }
964 break;
965 case 1:
966 //目標點是空位置,則炮無法隔一子移動
967 if (!cb.v_position[c][d]) {
968 return 1;
969 }
970 else {
971 //目標位置是己方棋子,則不能吃,返回1
972 if (cb.v_position[c][d]->getColor() == cb.v_position[a][b]->getColor()) {
973 return 1;
974 }
975 else {
976 return 3;
977 }
978 }
979 break;
980 default:
981 return 1;
982
983 }
984 }
985 }
986 }
987 //炮不走直線,不能移動,返回1
988 else {
989 return 1;
990 }
991 }
992 else {
993 return 1;
994 }
995 }
996
997 //judgePositionIsNULL
998 bool Player::judge(int a, int b)
999 {
1000 if (!cb.judgePositionIsNULL(a, b) && cb.judgePositionIsColor(a, b) == color)
1001 return true;
1002 else
1003 return false;
1004 }
1005 void Player::moveChess(int a, int b, int c, int d)
1006 {
1007 cb.v_position[c][d] = cb.v_position[a][b];
1008 cb.v_position[a][b] = NULL;
1009 }
1010 void Player::eatChess(int a, int b, int c, int d)
1011 {
1012 if (cb.v_position[c][d]->getName() == "帥" || cb.v_position[c][d]->getName() == "將")
1013 {
1014 cb.v_position[c][d] = cb.v_position[a][b];
1015 cb.v_position[a][b] = NULL;
1016 system("cls");
1017 cb.showBoard();
1018 if (cb.v_position[c][d]->getColor() == 1)
1019 cout << "紅棋方即上方玩家勝利" << endl;
1020 if (cb.v_position[c][d]->getColor() == 2)
1021 cout << "黑棋方即下方玩家勝利" << endl;
1022 system("pause");
1023 exit(0);
1024 }
1025 else
1026 {
1027 cb.v_position[c][d] = cb.v_position[a][b];
1028 cb.v_position[a][b] = NULL;
1029 }
1030 }
1031 void Player::inPut()
1032 {
1033 int a, b, c, d;
1034 cout << "輸入要移動的子位置坐標 先行數再列數 :";
1035 while (cin >> a >> b) {
1036 //判斷選擇點是否在棋盤界內
1037 if ((a >= 0 && a <= 9) && (b >= 0 && b <= 8) && judge(a, b)) {
1038 break;
1039 }
1040 system("cls");
1041 cb.showBoard();
1042 cout << "選擇位置不合法,請重新輸入:";
1043 }
1044 cout << "輸入目標位置:";
1045 while (cin >> c >> d) {
1046 //判斷選擇點是否在棋盤界內
1047 if ((a >= 0 && a <= 9) && (b >= 0 && b <= 8) && cb.v_position[a][b]->judge(a, b, c, d) != 1) {
1048 break;
1049 }
1050 system("cls");
1051 cb.showBoard();
1052 cout << "目標位置不合法 無法移動,請重新輸入:";
1053 }
1054 /*if (cb.v_position[a][b]->judge(a, b, c, d) == 1)
1055 {
1056 system("cls");
1057 cb.showBoard();
1058 cout << "目標位置不合法 無法移動" << endl;
1059 inPut();
1060 }*/
1061 if (cb.v_position[a][b]->judge(a, b, c, d) == 2)
1062 {
1063 moveChess(a, b, c, d);
1064 }
1065 else //(cb.v_position[a][b]->judge(a, b, c, d) == 3)
1066 {
1067 eatChess(a, b, c, d);
1068 }
1069 }
1070 int main()
1071 {
1072 Player player1(1);
1073 Player player2(2);
1074 cb.init_Red();
1075 cb.init_Black();
1076 cout << cb.v_position[0][2]->judge(0, 2, 2, 0);
1077
1078 while (1)
1079 {
1080 system("cls");
1081 cb.showBoard();
1082 cout << "上方玩家";
1083 player1.inPut();
1084 system("cls");
1085 cb.showBoard();
1086 cout << "下方玩家";
1087 player2.inPut();
1088 }
1089 return 0;
1090
1091 }
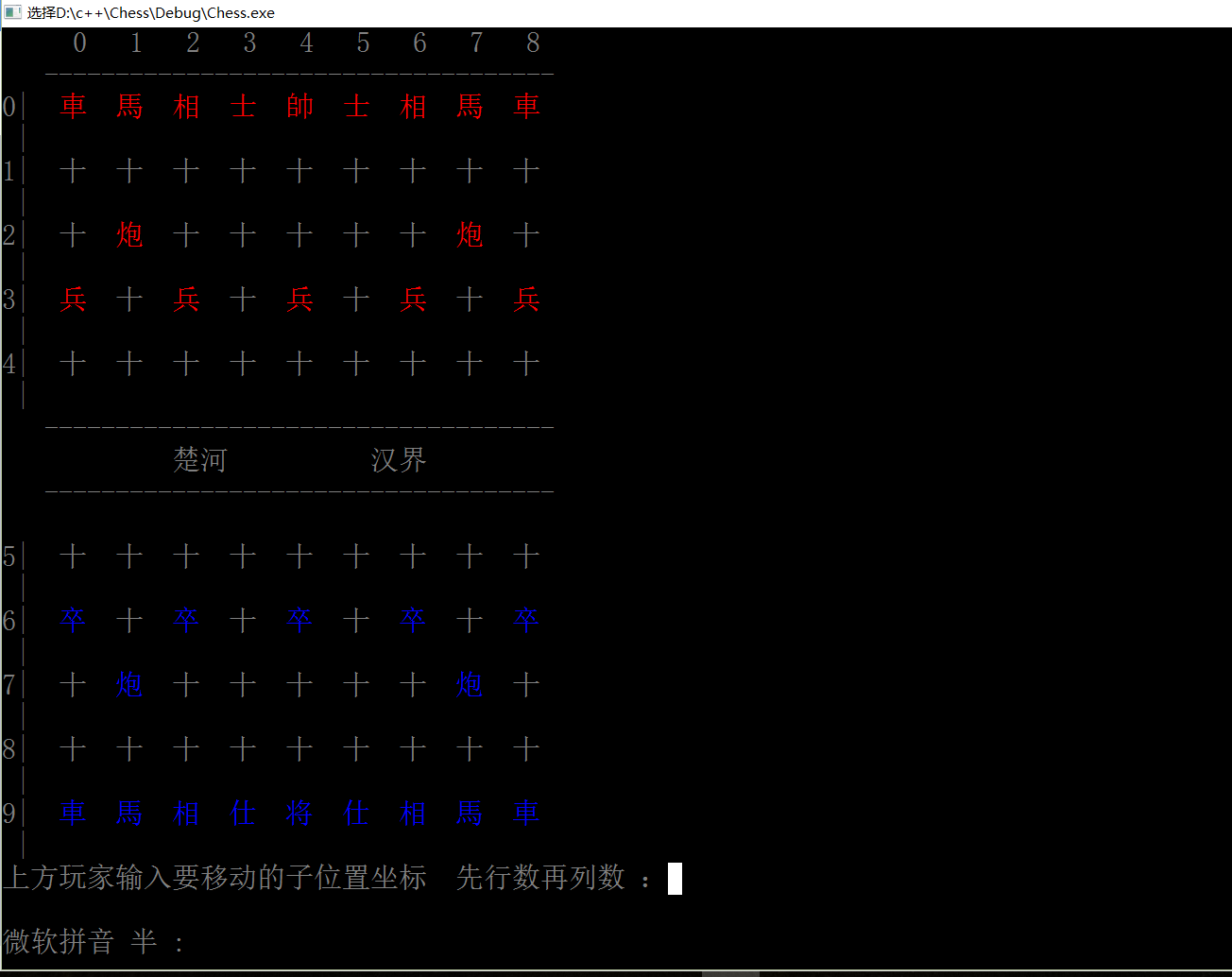
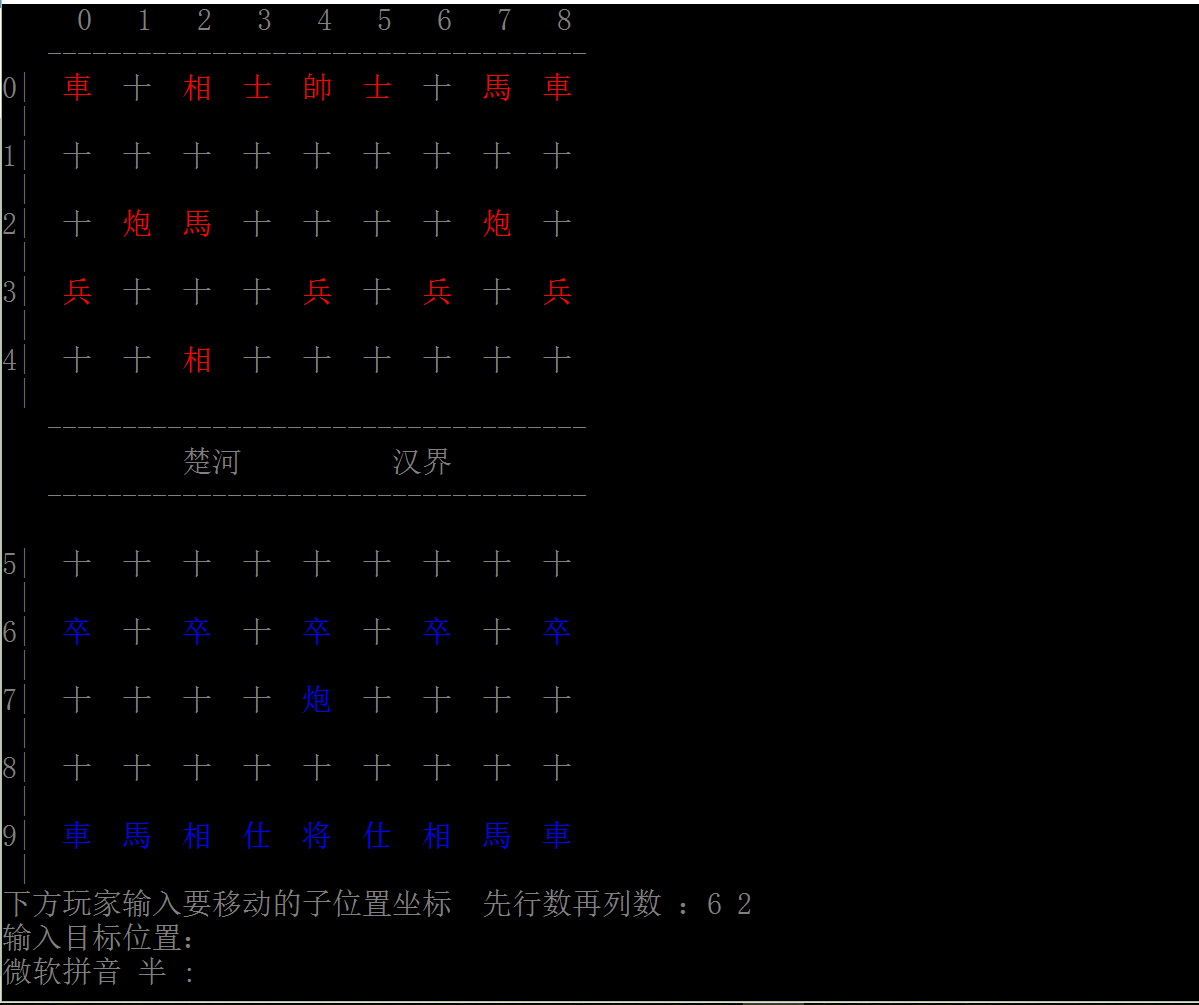
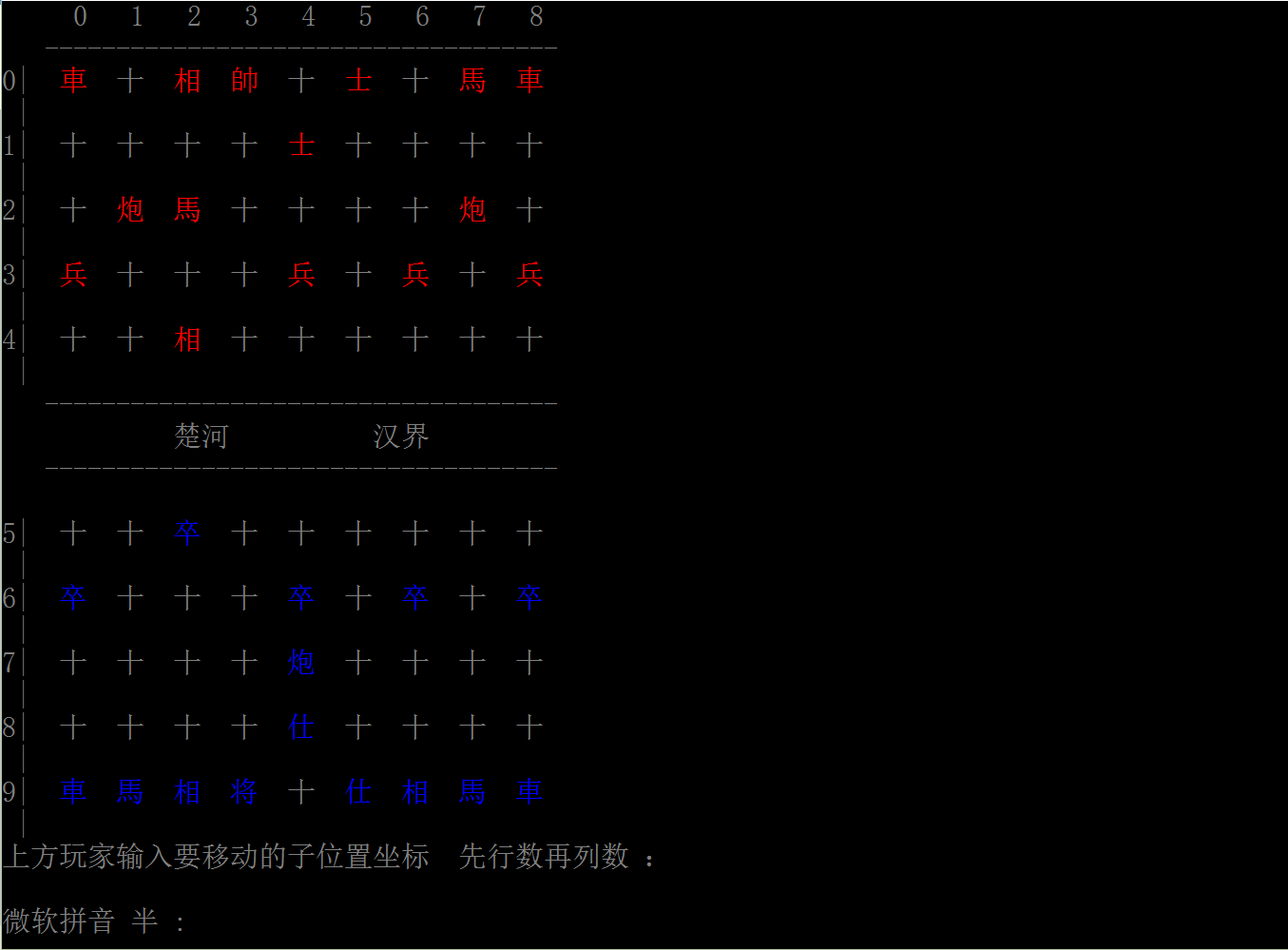