在看C++編程思想中,每個練習基本都是使用ofstream,ifstream,fstream,以前粗略知道其用法和含義,在看了幾位大牛的博文後,進行整理和總結:
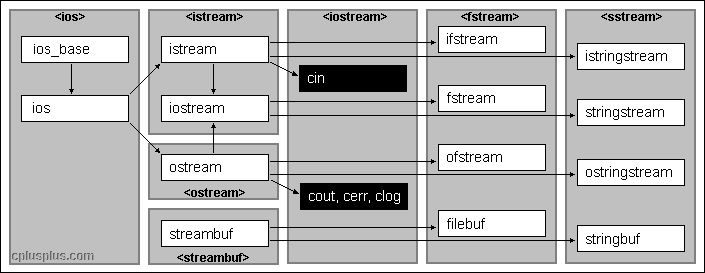
這裡主要是討論fstream的內容:
[java] view plaincopyprint?
- #include <fstream>
- ofstream //文件寫操作 內存寫入存儲設備
- ifstream //文件讀操作,存儲設備讀區到內存中
- fstream //讀寫操作,對打開的文件可進行讀寫操作
在fstream類中,成員函數open()實現打開文件的操作,從而將數據流和文件進行關聯,通過ofstream,ifstream,fstream對象進行對文件的讀寫操作
函數:open()
[cpp] view plaincopyprint?
- <span >
- public member function
-
- void open ( const char * filename,
- ios_base::openmode mode = ios_base::in | ios_base::out );
-
- void open(const wchar_t *_Filename,
- ios_base::openmode mode= ios_base::in | ios_base::out,
- int prot = ios_base::_Openprot);
-
- </span>
參數: filename 操作文件名
mode 打開文件的方式
prot 打開文件的屬性 //基本很少用到,在查看資料時,發現有兩種方式
打開文件的方式在ios類(所以流式I/O的基類)中定義,有如下幾種方式:
這些方式是能夠進行組合使用的,以“或”運算(“|”)的方式:例如
[cpp] view plaincopyprint?
- ofstream out;
- out.open("Hello.txt", ios::in|ios::out|ios::binary) //根據自己需要進行適當的選取
打開文件的屬性同樣在ios類中也有定義:
0
普通文件,打開操作
1
只讀文件
2
隱含文件
4
系統文件
對於文件的屬性也可以使用“或”運算和“+”進行組合使用,這裡就不做說明了。
很多程序中,可能會碰到ofstream out("Hello.txt"), ifstream in("..."),fstream
foi("...")這樣的的使用,並沒有顯式的去調用open()函數就進行文件的操作,直接調用了其默認的打開方式,因為在stream類的構造函數
中調用了open()函數,並擁有同樣的構造函數,所以在這裡可以直接使用流對象進行文件的操作,默認方式如下:
[cpp] view plaincopyprint?
- <span >
- ofstream out("...", ios::out);
- ifstream in("...", ios::in);
- fstream foi("...", ios::in|ios::out);
-
- </span>
當使用默認方式進行對文件的操作時,你可以使用成員函數is_open()對文件是否打開進行驗證
[cpp] view plaincopyprint?
- // writing on a text file
- #include <fiostream.h>
- int main () {
- ofstream out("out.txt");
- if (out.is_open())
- {
- out << "This is a line.\n";
- out << "This is another line.\n";
- out.close();
- }
- return 0;
- }
- //結果: 在out.txt中寫入:
- This is a line.
- This is another line
從文件中讀入數據也可以用與 cin>>的使用同樣的方法:
[cpp] view plaincopyprint?
- // reading a text file
- #include <iostream.h>
- #include <fstream.h>
- #include <stdlib.h>
-
- int main () {
- char buffer[256];
- ifstream in("test.txt");
- if (! in.is_open())
- { cout << "Error opening file"; exit (1); }
- while (!in.eof() )
- {
- in.getline (buffer,100);
- cout << buffer << endl;
- }
- return 0;
- }
- //結果 在屏幕上輸出
- This is a line.
- This is another line
- bad()
- fail()
- eof()
- good()
- tellg() 和 tellp()
- seekg() 和seekp()
seekp ( pos_type position );
seekp ( off_type offset, seekdir direction );
[cpp] view plaincopyprint?
- // obtaining file size
- #include <iostream.h>
- #include <fstream.h>
-
- const char * filename = "test.txt";
-
- int main () {
- long l,m;
- ifstream in(filename, ios::in|ios::binary);
- l = in.tellg();
- in.seekg (0, ios::end);
- m = in.tellg();
- in.close();
- cout << "size of " << filename;
- cout << " is " << (m-l) << " bytes.\n";
- return 0;
- }
-
- //結果:
- size of example.txt is 40 bytes.
read ( char * buffer, streamsize size );
[cpp] view plaincopyprint?
- // reading binary file
- #include <iostream>
- #include <fstream.h>
-
- const char * filename = "test.txt";
-
- int main () {
- char * buffer;
- long size;
- ifstream in (filename, ios::in|ios::binary|ios::ate);
- size = in.tellg();
- in.seekg (0, ios::beg);
- buffer = new char [size];
- in.read (buffer, size);
- in.close();
-
- cout << "the complete file is in a buffer";
-
- delete[] buffer;
- return 0;
- }
- //運行結果:
- The complete file is in a buffer
- 當文件被關閉時: 在文件被關閉之前,所有還沒有被完全寫出或讀取的緩存都將被同步。
- 當緩存buffer 滿時:緩存Buffers 有一定的空間限制。當緩存滿時,它會被自動同步。
- 控制符明確指明:當遇到流中某些特定的控制符時,同步會發生。這些控制符包括:flush 和endl。
- 明確調用函數sync(): 調用成員函數sync() (無參數)可以引發立即同步。這個函數返回一個int 值,等於-1 表示流沒有聯系的緩存或操作失敗。