比較字符串是指按照字典排序的規則,判斷兩個字符串的大小。前面的字母要小於後面的字母。String類中,常見的比較字符串的方法有Compare、CompareTo、CompareOrdinal以及Equals等。
各參數的含義如下:
strA,strB--待比較的兩個字符串;ignoreCase--指定是否考慮大小寫,當取true時忽略大小寫;indexA,indexB--需要比較兩個字符串的子串時,indexA和indexB分別為子字符串的起始位置;length--待比較字符串的最大長度;culture--字符串的區域性信息。
Compare方法的返回值:若strA>strB返回正整數; 若strA=strB,返回0; 若strA
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.IO;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "你好";
string strB = "你好嗎";
// 字符串比較
Console.WriteLine(string.Compare(strA, strB));
Console.WriteLine(string.Compare(strA, strA));
Console.WriteLine(string.Compare(strB, strA));
}
}
}
輸出:
vc/Bvbj219a3+7Suo7oKPGJyPgoKPHByZSBjbGFzcz0="brush:java;">string strA = "你好";
string strB = "你好嗎";
Console.WriteLine(strA.CompareTo(strB));
Equals方法
如果兩個字符串相等,Equals()返回值為true;否則,返回false。
string strA = "你好";
string strB = "你好嗎";
Console.WriteLine(string.Equals(strA, strB));
Console.WriteLine(strA.Equals(strB));
定位字符及子串
定位子串是指一個字符串中尋找其中包含的子串或者某個字符,String類中常見方法包括StartsWith/EndsWith、IndexOf/LastIndexOf 以及 IndexOfAny/LastIndexOfAny。
StartsWith/EndsWith
StartWith方法可以判斷一個字符串對象是否以另一個子字符串開頭,如果是,返回true。
Public bool StartsWith(String value);
其中,value表示待判定的子字符串。
EndsWith方法判斷一個字符串對象是否以另一個子字符串結尾。
IndexOf/LastIndexOf
IndexOf方法用於搜索在上一個字符串中,某個特定的字符或字符串第一次出現的位置,該方法區分大小寫,並從字符串的首字符開始以0計數。如果字符串中不包含這個字符或子串,則返回-1。IndexOf主要有以下重載形式:
定位字符
Int IndexOf(char value)
Int IndexOf(char value, int startIndex)
Int IndexOf(char value, int startIndex, int count)
定位子串
int IndexOf(string value)
int IndexOf(string value, int startIndex)
int IndexOf(string value, int startIndex, int count)
各參數的含義是:
value--帶定位的字符或子串;startIndex--在總串中開始搜索的起始位置;count--在總串中從起始位置開始搜索的字符數。
string strA = "Hello";
Console.WriteLine(strA.IndexOf('l'));
同IndexOf類似,LastIndexOf方法用於搜索在一個字符串中,某個特定的字符或子串最後一次出現的位置。
IndexOfAny/LastIndexOfAny
IndexOfAny方法功能與IndexOf類似,區別在於它可以在一個字符串中搜索一個字符數組中任意字符第一次出現的位置。同樣,該方法區分大小寫,並從字符串的首字符開始以0計數。如果字符串中不包含這個字符或子串,則返回-1。
IndexOfAny有以下重載形式:
int IndexOfAny(char[] anyOf)
int IndexOfAny(char[] anyOf, int startIndex)
int IndexOfAny(char[] anyOf, int startIndex, int count)
各參數的含義:
anyOf--待定位的字符數組,方法將返回這個數組中任意一個字符第一次出現的位置;startIndex--在總串中開始搜索的起始位置;count--在總串中從起始位置開始搜索的字符數。
string strA = "Hello";
char[] anyOf = { 'e', 'o' };
Console.WriteLine(Convert.ToString(strA.IndexOfAny(anyOf)));
Console.WriteLine(Convert.ToString(strA.LastIndexOfAny(anyOf)));
同IndexOfAny類似,LastIndexOfAny用於在一個字符串中搜索一個字符數組中任意字符最後一次出現的位置。
格式字符串
Format方法用於創建格式化的字符串以及連接多個字符串對象。最常用的重載形式為
public static string Format(string Format, params object[] arge);
format--用於指定返回的字符串的格式; args--一系列變量參數。
string newStr = string.Format("{0},{1}!!!", strA, strB);
在特定的應用中,Format方法也很方便。例如,將當前時間格式為"YYYY-MM-DD"形式:
DateTime DTA = DateTime.Now();
string strB = string.Format("{0:d}", DTA);
說明:{0:d}表示將時間格式化為短日期表示形式。
截取字符串
截取字符串需要用到String類的Substring方法,該方法用來從字符傳中檢索子字符串,有以下重載形式:
從字符串中檢索子字符串,子字符串從指定的字符位置開始
public string Substring(int startIndex)
startIndex--字符串中子字符串的起始字符位置。
返回值:一個String對象,等於字符串中從startIndex開始的子字符串,如果startIndex等於此字符串的長度,返回Empty。
從字符串中檢索子字符串,子字符串從指定的字符位置開始且具有指定的長度
public string Substring(int startIndex, int length)
startIndex--字符串中子字符串的起始字符位置。
length--子字符串中的字符數。
返回值:一個String對象,等於字符串中從startIndex開始的長度為length的子字符串,如果startIndex等於此字符串的長度,且length為0,返回Empty。
分隔字符串
使用Split方法可以將一個字符串,按照某個分隔符,分割成一系列小的字符串。
Split方法有多個重載形式,最常見的:
public string[] split(params char[] separator);
separator--一個數組,包含分隔符。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "Hello^^world";
char[] separator={'^'};
string[] splitstrings=new string[100];
splitstrings=strA.Split(separator);
int i= 0;
while(i 輸出:
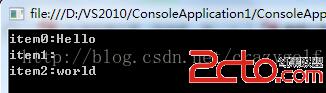
插入和填充字符串
String類可以用Insert方法在字符串的任意位置插入任意字符。而使用PadLeft/PadRight方法,可以在一個字符串的左右兩側可以進行字符填充。
Insert方法
Insert方法用於在一個字符串的指定位置插入另一個字符串,從而構造一個新的字符串。最常用的重載形式:
public string Insert(int startIndex, string value);
startIndex--用於指定要插入的位置,索引從0開始。
value--指定要插入的字符串。
返回值--指定字符串的一個新String等效項,但在位置startIndex處插入value。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "Hello^^world";
string strB = "Good morning!";
string newStr = strA.Insert(1, strB);
Console.WriteLine(newStr);
Console.ReadLine();
}
}
}
輸出:
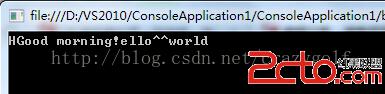
PadLeft/PadRight
PadLeft用於在一個字符串左側進行字符填充,使其達到一定的長度。有以下重載形式:
public string PadLeft(int totalWidth)
public string PadLeft(int totalWidth, char paddingChar)
totalWidth--指定填充後的字符長度。
paddingChar--指定要填充的字符,如果缺省,則填充空格符號。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "Hello^^world";
string newStr;
newStr = strA.PadLeft(16, '*');
Console.WriteLine(newStr);
Console.ReadLine();
}
}
}
輸出:
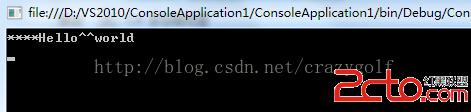
PadRight功能類似,不再贅述。
刪除和剪切字符串
String類使用Remove在字符串任意位置刪除任意長度子字符串,也可以使用Trim、TrimEnd和TrimStart剪切掉字符串中的一些特定字符串。
Remove方法
Remove方法從一個字符串的指定位置開始,刪除指定數量的字符。最常用的語法格式:
Public String Remove(int startIndex, int count);
startIndex--用於指定開始刪除的位置,索引從0開始。
count--指定刪除的字符數量。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "Hello^^world";
string newStr;
newStr = strA.PadLeft(16, '*');
Console.WriteLine(newStr);
Console.WriteLine(newStr.Remove(2, 3));
Console.ReadLine();
}
}
}
輸出:

Trim方法
有以下重載形式:
從字符串的開始位置和末尾移除空白字符的所有匹配項。
public string Trim()
返回值--一個新String,相當於將指定字符串首位空白字符移除後形成的字符串。
從字符串的開始位置和末尾移除數組中指定的一組字符的所有匹配項
public string Trim(params char[] trimChars)
trimChars--數組包含了指定要去掉的字符,如果缺省,則刪除空格符號)。
返回值--從指定字符串的開始和結尾移除trimChars中字符的所有匹配項後剩余的String。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "Hello^^world";
string newStr;
newStr = strA.PadLeft(16, '*');
Console.WriteLine(newStr);
char[] strB={'*','d'};
Console.WriteLine(newStr.Trim(strB));
Console.ReadLine();
}
}
}
輸出:

TrimStart方法
TrimStart方法用來從字符串的開始位置移除數組中指定的一組字符的所有匹配項。
public string TrimStart(params char[] trimChars)
trimChars--數組包含了指定要去掉的字符,如果缺省,則刪除空格符號)。
返回值--從指定字符串的開始位置移除trimChars中字符的所有匹配項後剩余的String。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "Hello^^world";
char[] strB = { 'H', 'o' };
string strC = strA.TrimStart(strB);
Console.WriteLine(strC);
Console.Read();
}
}
}
輸出:
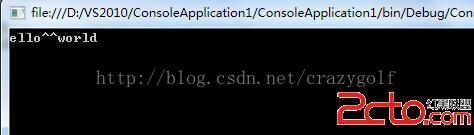
PS:注意字符區分大小寫。如上面改為"h",則仍然輸出Hello^^World。
TrimEnd方法
類比TrimStart即可。
復制字符串
String類包含了復制字符串的兩種方法Copy和CopyTo。
Copy方法
若要把一個字符串賦值到另一個字符數組中,可以使用String的靜態方法Copy來實現。
public static string Copy(string str);
str--為需要復制的源字符串,方法返回目標字符串。
返回值--與str具有相同值的新String。
CopyTo方法
CopyTo方法功能更為豐富,可以復制源字符串的一部分到一個字符數組中。另外,它不是靜態方法。
public void CopyTo(int sourceIndex, char[] destination, int destinationIndex, int count)
各參數的含義:
sourceIndex--需要復制的字符的起始位置。destination--目標字符數組。destinationIndex--指定目標數組的開始存放位置。count--指定要復制的字符個數。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "Hello^^world";
char[] strB = new char[100];
strA.CopyTo(3, strB, 0, 3);
Console.WriteLine(strB);
Console.Read();
}
}
}
輸出:
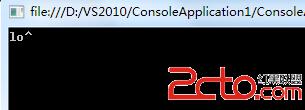
替換字符串
如果要替換掉某個字符串的某些特定字符或者某個子串,可以使用Repalce方法。
Replace方法
其語法形式主要有:public string Replace(char oldChar,char newChar)
public string Replace(string oldValue,string newValue)
oldChar--待替換的字符。 oldChar--待替換的子串。 newChar--替換後的新字符。 newValue--替換後的新子串。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
string strA = "Hello";
string strB=strA.Replace("ll","r");
Console.WriteLine(strB);
Console.Read();
}
}
}
輸出:
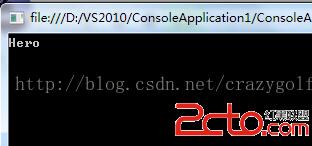
StringBuilder的定義和使用
StringBuilder類位於System.Text命名空間下,它表示可變字符串。
StringBuilder的定義
StringBuilder myStringBuilder=new StringBuilder();
StringBuilder的構造函數:
StringBuilder()--初始化StringBuilder類的新實例。StringBuilder(Int32)--使用指定的容量初始化StringBuilder類的新實例。StringBuilder(String)--使用指定的字符串初始化StringBuilder類的新實例。StringBuilder(Int32,Int32)--初始化StringBuilder類的新實例,該類起始於指定容量並且可增長到指定的最大容量。StringBuilder(String,Int32)--使用指定的字符串和容量初始化StringBuilder類的新實例。StringBuilder(String,Int32,Int32,Int32)--使用指定的子字符串和容量初始化StringBuilder類的新實例。
StringBuilder使用
StringBuilder類常用屬性說明:
Capacity--獲取或設置可包含在當前實例所分配的內存中的最大字符數。Chars--獲取或設置此實例中指定字符位置處的字符。Length--獲取或設置此實例的長度。MaxCapacity--獲取此實例的最大容量。
StringBuilder類常用的方法說明:
Append--在此實例的結尾追加指定對象的字符串表示形式。AppendFormat--向此實例追加包含零個或更多格式規范的格式化字符串。每個格式規范由相應對象的字符串表示形式替換。AppendLine--將默認的行終止符(或指定字符串的副本和默認的行終止符)追加到此實例的結尾。CopyTo--將此實例的指定段中的字符復制到目標Char數組的指定段中。EnsureCapacity--將確保StringBuilder的此實例的容量至少是指定值。Insert--將指定對象的字符串表示形式插入到此實例中的指定字符位置。Remove--將指定范圍的字符從此實例中移除。Replace--將此實例中所有的指定字符或字符串替換為其他的指定字符或字符串。ToString--將StringBuilder的值轉化為String。
StringBuilder類表示值為可變字符序列的對象,其值可變的原因是同過追加、刪除、替換或插入字符而創建後的實例可以對其進行修改。幾乎所有修改此類的實例方法都返回對同一實例的引用。
StringBuilder的容量是實例在任何給定時間可存儲的最大字符數,並且大於或等於實例值的字符串表示形式的長度。容量可以通過Capacity屬性或EnsureCapacity方法來增加或減少,但不能小於Length屬性的值。
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
StringBuilder Builder = new StringBuilder("Hello", 100);
Builder.Append(" World");
Console.WriteLine(Builder);
Builder.AppendFormat("{0} End", "@");
Console.WriteLine(Builder);
Builder.AppendLine("This is one line.");
Console.WriteLine(Builder);
Builder.Insert(0, "Begin");
Console.WriteLine(Builder);
Builder.Remove(19, Builder.Length - 19);
Console.WriteLine(Builder);
Builder.Replace("Begin", "Begin:");
Console.WriteLine(Builder);
Console.ReadLine();
}
}
}
輸出:
系統開銷可能非常昂貴。如果要修改字符串而不創建新的對象,請使用StringBuilder類,避免產生過多臨時對象。