在平時比賽的時候,我們在Excel裡面寫入數據後,需要排名還要進行按鈕的點擊,小王覺得有點煩,作為強大的編程語言Python,難道不可以嗎?答案是,當然可以!
項目說明:程序運行後,提示用戶有選項菜單,用戶根據菜單進行成績錄入,簡單方便,隨時查看!
涉及知識:元組列表,條件語句,循環,字符串,簡單算法,字典,函數
元組算法
# -*- coding : utf-8 -*-
# @Time : 2020/8/8
# @author : 王小王
# @Software : PyCharm
# @CSDN : https://blog.csdn.net/weixin_47723732
scores = []
choice = None
print('''
成績錄入小程序
0 - 結束程序
1 - 輸入姓名成績並錄入
2 - 打印排名好後的成績
3 - 查找特定學生的成績
4 - 刪除特定學生的成績
''')
while choice != "0":
choice = input("Choice: ")
if choice == "0":
print("結束程序!")
elif choice == "1":
name = input("輸入姓名: ")
score = int(input("輸入成績: "))
listing = (score, name)
scores.append(listing)
scores.sort(reverse=True)
elif choice == "2":
print(" 成績排名")
print("姓名\t成績")
for listing in scores:
score, name = listing
print(name, "\t", score)
elif choice == "3":
Name = input("輸入你要查找的姓名:")
for each in scores:
if each[1] == Name:
score ,name=each
print("姓名\t成績")
print(name, "\t", score )
elif choice == "4":
Name = input("輸入您要刪除成績的姓名: ")
for each in scores:
if each[1] == Name:
scores.remove(each)
else:
print("你輸入的選擇有誤,請檢查!")
input("\n\nPress the enter key to exit.")
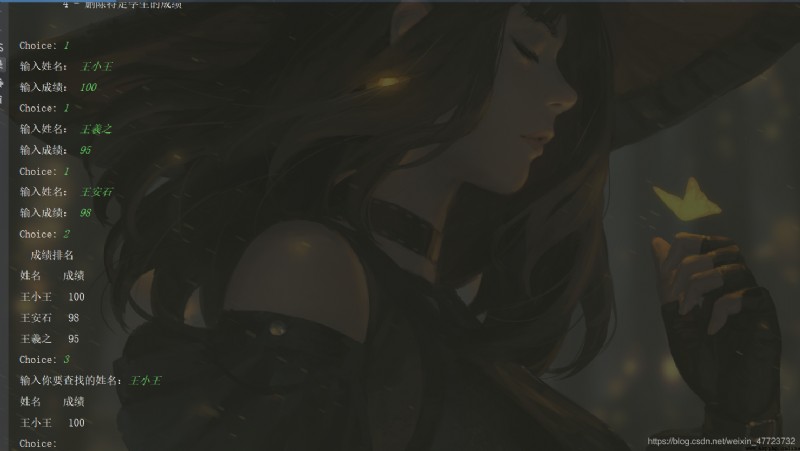
字典算法
# -*- coding : utf-8 -*-
# @Time : 2020/8/8
# @author : 王小王
# @Software : PyCharm
# @CSDN : https://blog.csdn.net/weixin_47723732
# 所有學生數據保存到一個列表,每個學生的信息用一個字典表示[{},{}]
records = []
def print_menu():
"""
打印出整個菜單
:return:
"""
print("""
學生信息系統
0 - 退出
1 - 顯示所有學生信息
2 - 添加學生信息
3 - 查找學生信息
4 - 刪除學生信息
5 - 排序
""")
def user_choice():
"""
獲取用戶輸入,並確保輸入數據在0-4之間
:return: 返回合法的用戶選擇
"""
choice = input("請選擇(0~5):")
# 確保用戶的選擇是在0~4
while int(choice) > 5 or int(choice) < 0:
choice = input("請重新選擇(0~5):")
return choice
def add_record():
"""
添加用戶數據
:return:
"""
name = input('請輸入學生的名字:')
while name == '':
name = input('請輸入學生的名字:')
# 確定學生信息是否已經錄入
for info in records:
if info['name'] == name:
print("該學生已經存在")
break
else:
score = float(input('請輸入成績(0~100):'))
while score < 0 or score > 100:
score = float(input('請輸入成績(0~100):'))
info = {
'name': name,
'score': score
}
records.append(info)
def display_records():
"""
展示數據
:return:
"""
print("所有學生信息")
for info in records:
print("姓名:", info['name'], '成績:', info['score'])
def search_record():
"""
根據學生名字查找信息,並輸出學生的成績
:return:
"""
name = input('請輸入學生的名字:')
while name == '':
name = input('請輸入學生的名字:')
for info in records:
if info['name'] == name:
print("學生成績為:", info['score'])
break
else:
print("沒有該學生")
def del_record():
"""
根據學生名字刪除數據
:return:
"""
name = input('請輸入學生的名字:')
while name == '':
name = input('請輸入學生的名字:')
for info in records:
if info['name'] == name:
records.remove(info)
break
else:
print("沒有該用戶")
choice = None
while choice != "0":
# 打印菜單
print_menu()
# 獲取用戶輸入
choice = user_choice()
# 用條件分支去判定各種選擇
if choice == '1':
# 顯示數據
display_records()
elif choice == '2':
# 添加一個學生數據
add_record()
elif choice == '3':
# 查找信息
search_record()
elif choice == '4':
# 刪除數據
del_record()
elif choice == '5':
records.sort(key=lambda item: item['score'])
elif choice == '0':
# 退出程序
print('歡迎下次登錄本系統')
else:
print('選擇錯誤!!!')
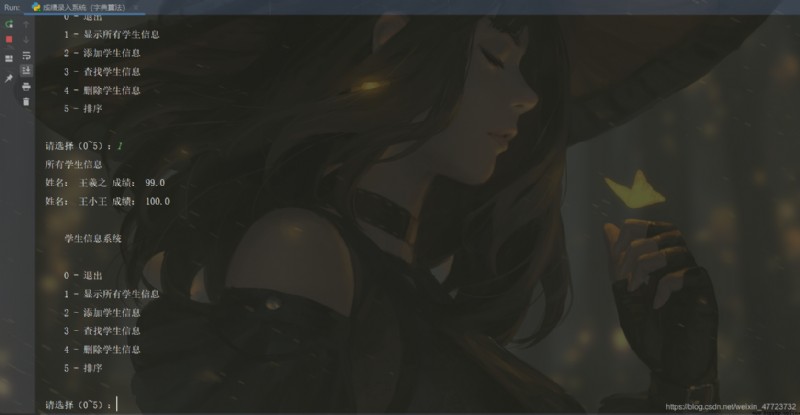
實際操作你們可以自己去試試!