Now we'll turn to the client side, and add a page that can consume data from the Admin controller. The page will allow users to create, edit, or delete products, by sending AJAX requests to the controller.
現在我們轉入客戶端,並添加一個能夠使用從Admin控制器而來的數據的頁面。通過給控制器發送AJAX請求的方式,該頁面將允許用戶創建、編輯,或刪除產品。
In Solution Explorer, expand the Controllers folder and open the file named HomeController.cs. This file contains an MVC controller. Add a method named Admin:
在“解決方案資源管理器”中,展開Controllers文件夾,並打開名為HomeController.cs的文件。這個文件是一個MVC控制器。添加一個名稱為Admin的方法:
復制代碼 代碼如下:
public ActionResult Admin()
{
string apiUri= Url.HttpRouteUrl("DefaultApi", new { controller = "admin", });
ViewBag.ApiUrl = new Uri(Request.Url, apiUri).AbsoluteUri.ToString();
return View();
}
The HttpRouteUrl method creates the URI to the web API, and we store this in the view bag for later.
HttpRouteUrl方法創建了發送給Web API的URI,我們隨後把它存儲在視圖包(view bag)中。
Next, position the text cursor within the Admin action method, then right-click and select Add View. This will bring up the Add View dialog.
下一步,把文本光標定位到Admin動作方法的內部,然後右擊,並選擇“添加視圖”。這會帶出“添加視圖”對話框(見圖2-20)。
圖2-20. 添加視圖
In the Add View dialog, name the view "Admin". Select the check box labeled Create a strongly-typed view. Under Model Class, select "Product (ProductStore.Models)". Leave all the other options as their default values.
在“添加視圖”對話框中,將此視圖命名為“Admin”。選中標簽為“創建強類型視圖”的復選框。在“模型類”下面,選擇“Product (ProductStore.Models)”。保留所有其它選項為其默認值(如圖2-21)。
圖2-21. “添加視圖”對話框的設置
Clicking Add adds a file named Admin.cshtml under Views/Home. Open this file and add the following HTML. This HTML defines the structure of the page, but no functionality is wired up yet.
點擊“添加”,會把一個名稱為Admin.cshtml的文件添加到Views/Home下。打開這個文件,並添加以下HTML。這個HTML定義了頁面的結構,但尚未連接功能。
復制代碼 代碼如下:
<div class="content">
<div class="float-left">
<ul id="update-products">
<li>
<div><div class="item">Product ID</div><span></span></div>
<div><div class="item">Name</div> <input type="text" /></div>
<div><div class="item">Price ($)</div> <input type="text" /></div>
<div><div class="item">Actual Cost ($)</div> <input type="text" /></div>
<div>
<input type="button" value="Update" />
<input type="button" value="Delete Item" />
</div>
</li>
</ul>
</div>
<div class="float-right">
<h2>Add New Product</h2>
<form id="product">
@Html.ValidationSummary(true)
<fieldset>
<legend>Contact</legend>
@Html.EditorForModel()
<p>
<input type="submit" value="Save" />
</p>
</fieldset>
</form>
</div>
</div>
Create a Link to the Admin Page
創建到Admin頁面的鏈接
In Solution Explorer, expand the Views folder and then expand the Shared folder. Open the file named _Layout.cshtml. Locate the ul element with id = "menu", and an action link for the Admin view:
在“解決方案資源管理器”中,展開Views文件夾,然後展開Shared文件夾。打開名稱為_Layout.cshtml的文件。定位到id = "menu"的ul元素,和一個用於Admin視圖的動作鏈接:
復制代碼 代碼如下:
<li>@Html.ActionLink("Admin", "Admin", "Home")</li>
In the sample project, I made a few other cosmetic changes, such as replacing the string “Your logo here”. These don't affect the functionality of the application. You can download the project and compare the files.
在這個例子項目中,我做了幾個其它裝飾性的修改,如替換了字符串“Your logo here(這是你的logo)”。這些不會影響此應用程序的功能。你可以下載這個項目並比較此文件。
Run the application and click the “Admin” link that appears at the top of the home page. The Admin page should look like the following:
運行該應用程序,並點擊出現在首頁頂部的這個“Admin”鏈接。Admin頁面看上去應當像這樣(見圖2-22):
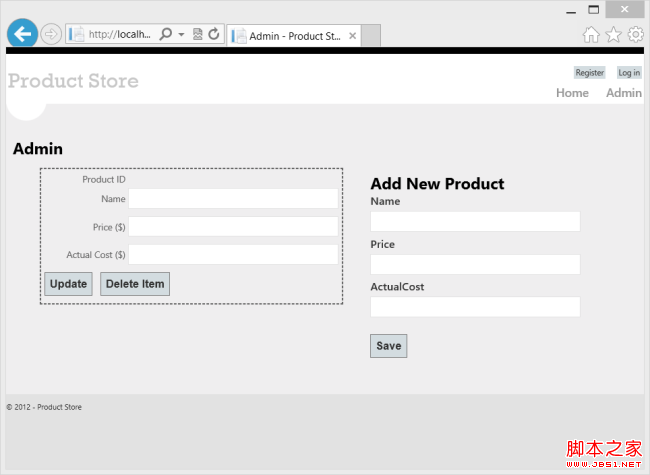
圖2-22. Admin頁面
Right now, the page doesn't do anything. In the next section, we'll use Knockout.js to create a dynamic UI.
此刻,這個頁面不做任何事情。在下一小節中,我們將使用Knockout.js來創建一個動態UI。
Add Authorization
添加授權
The Admin page is currently accessible to anyone visiting the site. Let's change this to restrict permission to administrators.
Admin此刻可以被任何訪問網站的人所訪問。讓我們做點修改,把許可限制到管理員。
Start by adding an "Administrator" role and an administrator user. In Solution Explorer, expand the Filters folder and open the file named InitializeSimpleMembershipAttribute.cs. Locate the SimpleMembershipInitializer constructor. After the call to WebSecurity.InitializeDatabaseConnection, add the following code:
先從添加“Administrator(管理員)”角色和administrator用戶開始。在“解決方案資源管理器”中,展開Filters文件夾,並打開名稱為InitializeSimpleMembershipAttribute.cs的文件,定位到SimpleMembershipInitializer構造器。在對WebSecurity.InitializeDatabaseConnection的調用之後,添加以下代碼:
復制代碼 代碼如下:
const string adminRole = "Administrator";
const string adminName = "Administrator";
if (!Roles.RoleExists(adminRole))
{
Roles.CreateRole(adminRole);
}
if (!WebSecurity.UserExists(adminName))
{
WebSecurity.CreateUserAndAccount(adminName, "password");
Roles.AddUserToRole(adminName, adminRole);
}
This is a quick-and-dirty way to add the "Administrator" role and create a user for the role.
這是添加“Administrator”角色並為該角色創建用戶的一種快速而直接的方式。
In Solution Explorer, expand the Controllers folder and open the HomeController.cs file. Add the Authorize attribute to the Admin method.
在“解決方案資源管理器”中,展開Controllers文件夾,並打開HomeController.cs文件。把Authorize(授權)注解屬性添加到Admin方法上:
復制代碼 代碼如下:
[Authorize(Roles="Administrator")]
public ActionResult Admin()
{
return View();
}Open the AdminController.cs file and add the Authorize attribute to the entire AdminController class.
打開AdminController.cs文件,並把Authorize注解屬性添加到整個AdminController類上:
[Authorize(Roles="Administrator")]
public class AdminController : ApiController
{
// ...
MVC and Web API both define Authorize attributes, in different namespaces. MVC uses System.Web.Mvc.AuthorizeAttribute, while Web API uses System.Web.Http.AuthorizeAttribute.
MVC和Web API都定義了Authorize注解屬性,但位於不同的命名空間。MVC使用的是System.Web.Mvc.AuthorizeAttribute,而Web API使用System.Web.Http.AuthorizeAttribute。
Now only administrators can view the Admin page. Also, if you send an HTTP request to the Admin controller, the request must contain an authentication cookie. If not, the server sends an HTTP 401 (Unauthorized) response. You can see this in Fiddler by sending a GET request to http://localhost:port/api/admin.
現在,只有管理員才可以查看Admin頁面。而且,如果對Admin控制器發送一個HTTP請求,該請求必須包含一個認證cookie。否則,服務器會發送一個HTTP 401(未授權)響應。在Fiddler中,通過發送一個http://localhost:port/api/admin的GET請求,便會看到這種情況。