Preface
The singleton pattern Maybe the simplest design pattern , Singletons are very generic objects . Allows you to guarantee that a class has only one instance , And provide a global node to access the instance .
We can think of the captain of a ship as a real-life example of the singleton model . On board , He is in charge . He is responsible for important decisions , Because of this responsibility , He received some requests .
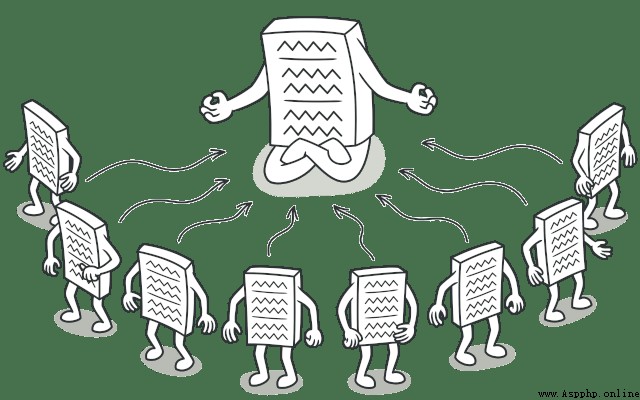
As mentioned earlier , One use case of the singleton pattern is to create a single object that maintains the global state of the program . Other possible use cases are as follows :
- Control concurrent access to shared resources ; for example , Object classes that manage connections to databases
- Horizontal services or resources , Because it can be accessed from different parts of the application or by different users to complete its work ; for example , Japan Log recording system Or utility The core class
class Singleton:
"""Definition of a Singleton object."""
singleton_instance = None
def __init__(self):
"""
Override the initialization
mechanism, returning only the single instance.
"""
...
@staticmethod
def get_singleton():
"""
Method for fetching the Singleton instance.
Is static so that it can be accessed everywhere.
"""
...
@staticmethod
def update_singleton(val):
"""
Method for setting value of Singleton instance.
Is static so that it can be accessed everywhere.
"""
...
Stored in Singleton The data in the instance is arbitrary . It is important to , Regardless of the data , What about the caller and the range ,Singleton Object will return the same instance . This makes the unit useful in implementing things such as global settings or run configuration .
Single example
Use the following code snippet to play Active Singleton Realization . Try using data structures ( For example, a dictionary ) Replace variable Singleton_instance, And look at Getter and Setter How to change the implementation of . Try writing some shares Singleton Function of instance .
class Singleton:
"""Definition of a Singleton object."""
# Maintain state of Singleton
singleton_instance = None
def __init__(self):
"""Override the initialization mechanism."""
if Singleton.singleton_instance is None:
Singleton.singleton_instance = self
@staticmethod
def get_singleton():
"""
Method for fetching the Singleton instance.
Is static so that it can be accessed everywhere.
"""
if Singleton.singleton_instance is None:
Singleton() # Call __init__ to initialize instance
return Singleton.singleton_instance
@staticmethod
def update_singleton(val):
"""
Method for setting value of Singleton instance.
Is static so that it can be accessed everywhere.
"""
if Singleton.singleton_instance is None:
Singleton() # Call __init__ to initialize instance
Singleton.singleton_instance = val
Singleton.update_singleton("Michael")
print("Value in Singleton instance is: " + Singleton.get_singleton())
Singleton() # Try to create a new Singleton instance
print("Value in Singleton instance is STILL: " + Singleton.get_singleton())
The singleton pattern can also be implemented by making singleton classes use metaclasses ( Its type , Has a previously defined metaclass ) To achieve . According to need , Metaclass
__call__()
Method to ensure that only one instance of a class can be created :
class SingletonMeta(type):
"""
The Singleton class can be implemented in different ways in Python. Some
possible methods include: base class, decorator, metaclass. We will use the
metaclass because it is best suited for this purpose.
"""
_instances = {}
def __call__(cls, *args, **kwargs):
"""
Possible changes to the value of the `__init__` argument do not affect
the returned instance.
"""
if cls not in cls._instances:
instance = super().__call__(*args, **kwargs)
cls._instances[cls] = instance
return cls._instances[cls]
class Singleton(metaclass=SingletonMeta):
def some_business_logic(self):
"""
Finally, any singleton should define some business logic, which can be
executed on its instance.
"""
# ...
if __name__ == "__main__":
# The client code.
s1 = Singleton()
s2 = Singleton()
if id(s1) == id(s2):
print("Singleton works, both variables contain the same instance.")
else:
print("Singleton failed, variables contain different instances.")
Advantages and disadvantages of singleton mode
advantage :
- You can guarantee that there is only one instance of a class .
- You get a global access node to the instance .
- Initialize singleton objects only when they are first requested .
shortcoming :
- A violation of the Principle of single responsibility . This model solves two problems at the same time .
- Singleton patterns can mask bad design , For example, each component of the program knows too much about each other .
- This mode needs special processing in multithreading environment , Avoid multiple threads creating singleton objects multiple times .
- Unit testing of singleton client code can be difficult , Because many test frameworks create mock objects in an inheritance based way . Because the constructor of the singleton class is private , And most languages can't override static methods , So you need to think of ways to simulate singletons carefully . Or don't write test code at all , Or don't use singleton mode .
Reference link :