定義
原型模式(Prototype Pattern)是一種創建型設計模式, 使你能夠復制已有對象, 而又無需使代碼依賴它們所屬的類。原型模式能夠讓我們利用克隆技術在現有對象的基礎上創建對象。
說到克隆,一個著名的非技術性例子是名為多莉的綿羊,它是由蘇格蘭的研究人員通過克隆乳腺中的一個細胞創造的。
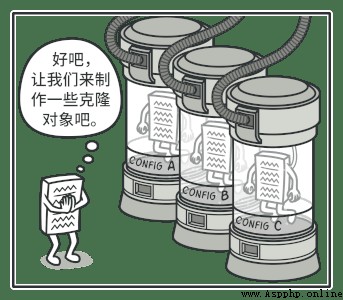
許多 Python 應用程序都使用了原型模式,但它很少被稱為原型,因為克隆對象是語言的一個內置功能。
原型模式的想法是使用該對象的完整結構的拷貝來產生新的對象。我們將看到,這在 Python 中幾乎是自然而然的,因為我們有一個拷貝功能,對使用這種技術有很大幫助。在創建一個對象的拷貝的一般情況下,所發生的是你對同一個對象做一個新的引用,這種方法叫做淺拷貝。但如果你需要復制對象,也就是原型的情況,你要做一個深拷貝。
實現
原型模式建議創建一個接口來創建現有對象的克隆。任何客戶都可以依賴的對象。Python 中可以通過類來進行實例化:
- 原型類:一個超類,它將包含對象克隆將具有的所有必要屬性和方法。此外,Prototype 有一個抽象的 clone() 方法,它必須由所有子類實現。
- 具體類:一旦我們創建了原型超類,我們就可以開始基於超類定義具體類。具體類是可選的,可以在應用程序中定義。
具體的類可以有自己的屬性和方法,但它們總是有原始的原型屬性和被覆蓋的
clone()
版本。
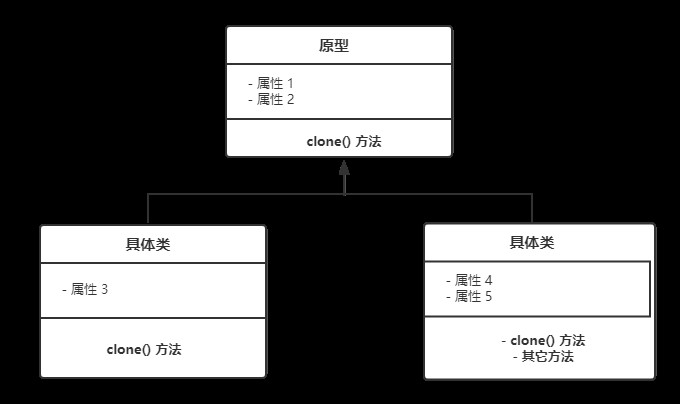
在 Python 中,考慮為 Car 對象創建一個原型。讓我們為汽車創建一個接口:
class Car:
def __init__(self, engine="1500cc", color="D-white", seats=7):
self.engine = engine
self.color = color
self.seats = seats
def __str__(self):
return f"{self.engine} | {self.color} | {self.seats}"
原型類:
- RegisterObject 方法在字典中添加元素,以新對象的名稱為鍵,以現有對象為值。
- DeregisterObject 方法從字典中刪除條目。
- 並且,最後通過 Clone 方法復制現有對象。克隆方法使用來自 Copy 模塊的 deepcopy() 方法來克隆對象。
import copy
class Prototype:
def __init__(self):
"""Dictionary that will stores cloned objects."""
self._ClonedObjects = {}
def RegisterObject(self, name, obj):
"""Method to store all clones of the existion objects."""
self._ClonedObjects[name] = obj
def DeregisterObject(self, name):
"""Method to delete the cloned object from the dictionary."""
del self._ClonedObjects[name]
def Clone(self, name, **kwargs):
"""Method to clone the object."""
clonedObject = copy.deepcopy(self._ClonedObjects.get(name))
clonedObject.__dict__.update(kwargs)
return clonedObject
最後,我們利用
main
函數來測試:
if __name__ == "__main__":
"""The object that will be cloned."""
defaultCar = Car()
prototype = Prototype()
"""The object that will be cloned."""
CarType1 = Car("1000cc", "Red", 4)
"""Registering the defaultCar in dictionary with its key as 'basicCar'"""
prototype.RegisterObject('BasicCar', defaultCar)
prototype.RegisterObject('Type-1', CarType1)
carOne = prototype.Clone('BasicCar', color = "Lake side brown")
carTwo = prototype.Clone('Type-1',color = "Red")
carThree = prototype.Clone('Type-1', color = "Moon Dust Silver")
print("Details of the default-car:", defaultCar)
print("Details of car-One:", carOne)
print("Details of car-Two:", carTwo)
print("Details of car-Three:", carThree)
運行輸出:
$ python protype.py
Details of the default-car: 1500cc | D-white | 7
Details of car-One: 1500cc | Lake side brown | 7
Details of car-Two: 1000cc | Red | 4
Details of car-Three: 1000cc | Moon Dust Silver | 4
總結
原型是一種創建型設計模式, 使你能夠復制對象, 甚至是復雜對象, 而又無需使代碼依賴它們所屬的類。
所有的原型類都必須有一個通用的接口, 使得即使在對象所屬的具體類未知的情況下也能復制對象。 原型對象可以生成自身的完整副本, 因為相同類的對象可以相互訪問對方的私有成員變量。
原型模式減少了子類的數量,隱藏了創建對象的復雜性,並便於在運行時添加或刪除對象。
參考鏈接:
- The Prototype Design Pattern in Python